How to Add Google Log-in Feature in Your App for Easy Registration Process
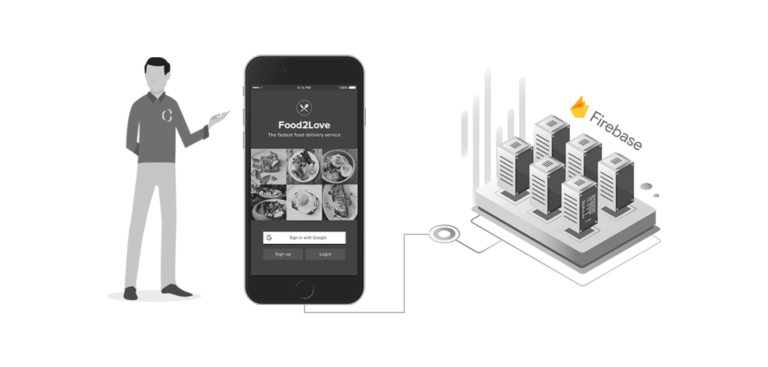
In this era of mobile and tablets, each of the company, be it food, banking or even gaming, have their own apps in the market. To thrive in this competition, every company is bent towards building their own applications. Logging in into these apps is made simple by either using google or Facebook logins.
The visitors, who wish to use the apps tend to avoid the huge data filling forms and sharing unnecessary information. For them, using a google or Facebook login is a convenient way to sign in the app. They just need to choose an option and within a fraction of seconds, they are right into the app environment, ready to explore more.
Opting for a google login has many benefits for the user. Faster registration is a major advantage which surpasses all other advantages. However, there are a lot more benefits than just considering the time factor.
» A social login can add value if a user is signing up for social functionality.
» The user is spared from remembering an extra password.
» It also allows a user to login with multiple identities to protect the original identity.
» The user gets a personalized experience.
» It is way too easier for smartphones.
Do you want to add the Google login for your mobile app? I have got it all covered for you.
How to add Firebase Google Login For Authentication.
1. Follow the below steps to do this:
1 » Visit the Firebase Website firebase.google.com and create an account to start with. Go to Firebase console and click the “Create New Project” Button.
2 » Create project window appears and you need to enter a project name and your Country/Region, Click Create Project to proceed.
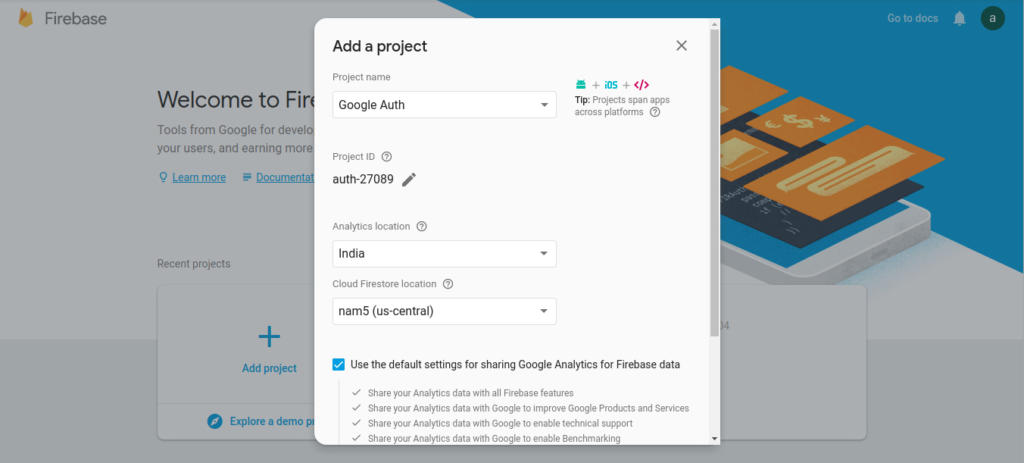
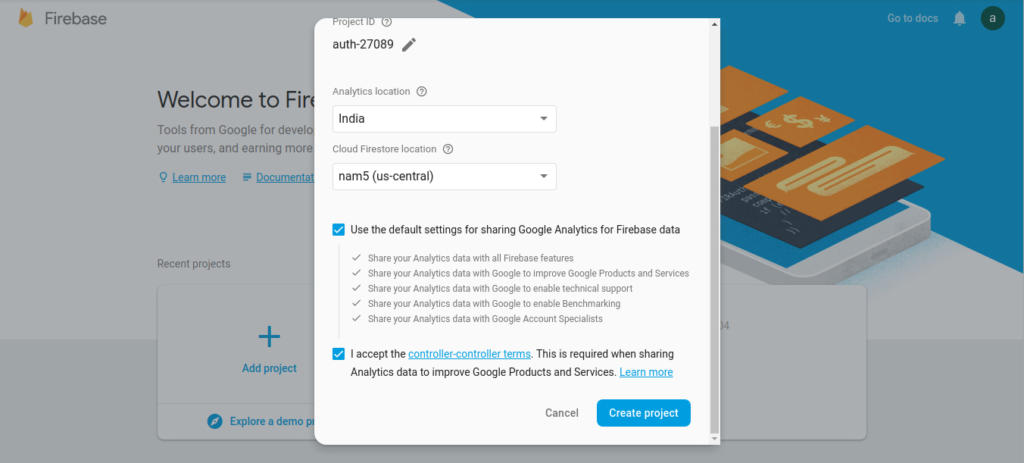
3 » In the next screen choose “Add Firebase to your Android App” and then add the package details and Debug signing certificate SHA-1 key. Click here for a tutorial on Getting Android Debug Keystore SHA1 Fingerprint:
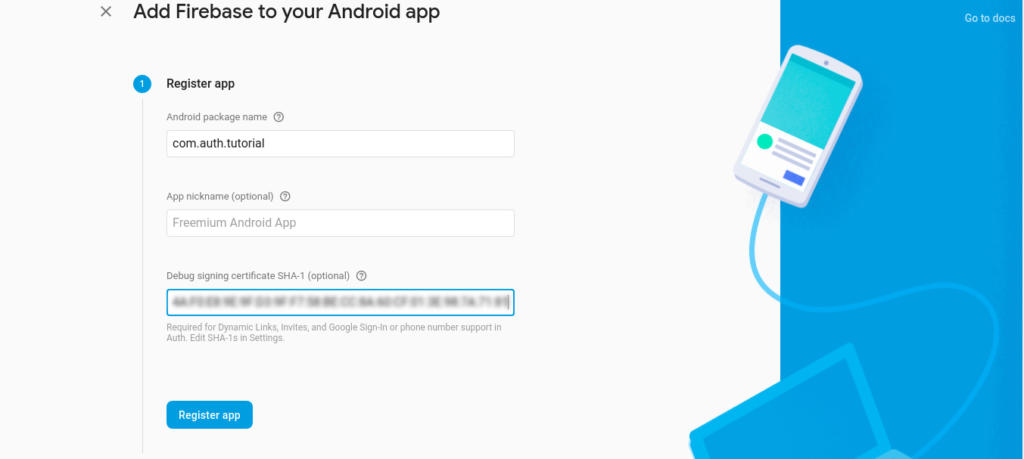
4 » After clicking Register App , you will be asked to download the google-services.json file. Please, download it to your computer. We will add it to our android app later.
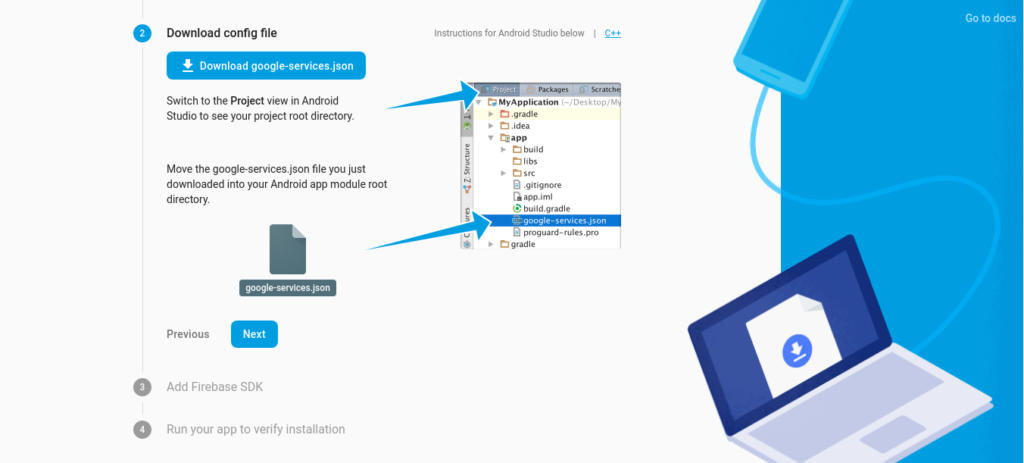
5 » After downloading the google-services.json file, you will be assisted on how to add “Firebase Sdk” to an android project. Now click on next to finish the project setup on Firebase console. Firebase will try to check the if the app has communicated with Firebase Server. You can simply click on Skip this step, it will take you to Firebase project Dashboard.
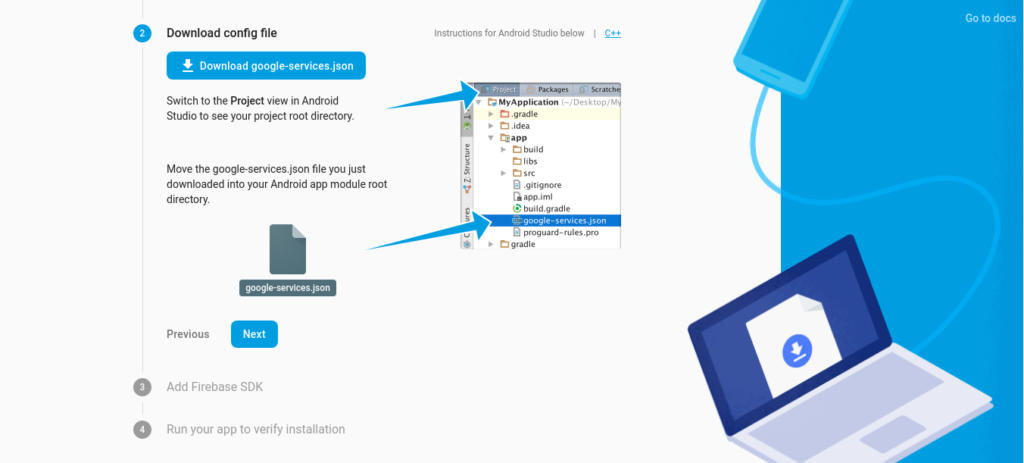
6 » In the project Dashboard, click the Develop Menu on the left of the page, then click the Authentication in the sub-Menu of the Develop.
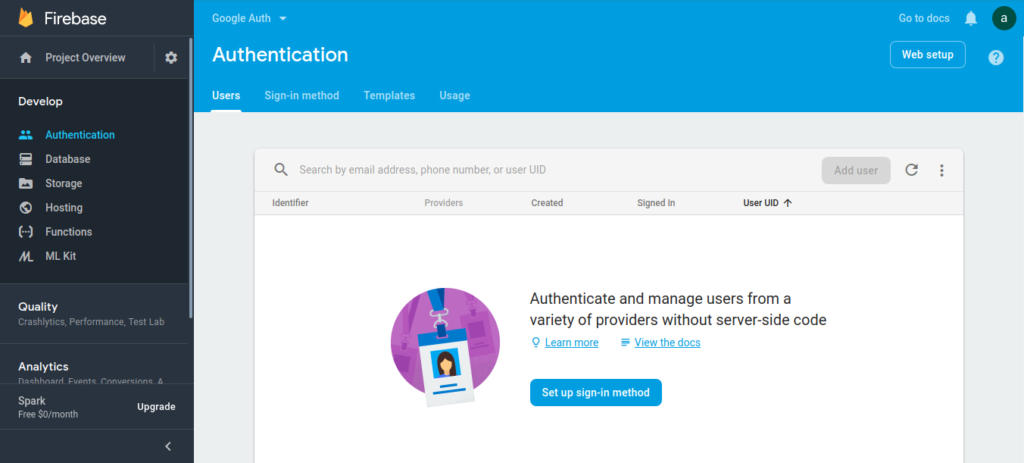
7 » Now you need to click on the Sign_In method Tab from where you can enable and disable different SignIn providers that Firebase Support, go ahead and Enable Google SignIn and then click save.
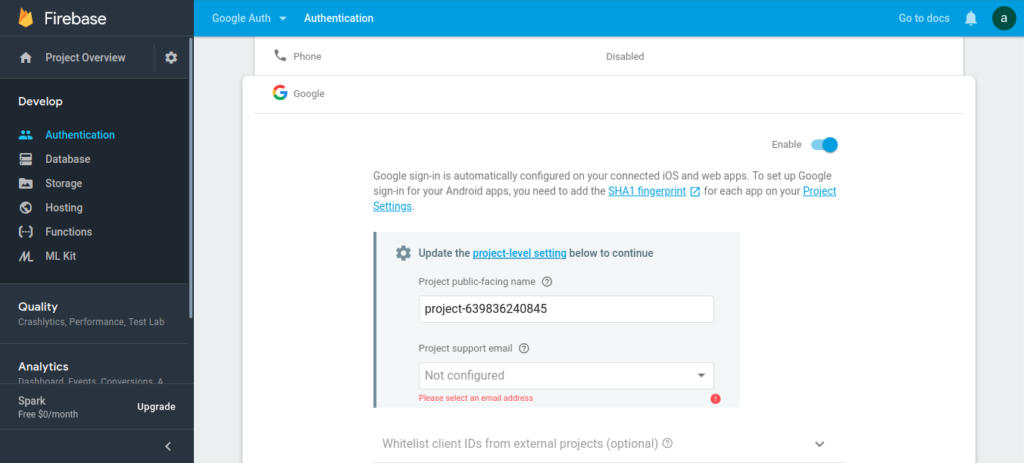
2. Create Android Firebase Login App in Android Studio.
Let’s create the Android Application that will connect to Firebase for user authentication using Firebase Google Login.
Steps to creating a project on Android Studio
» Launch Android Studio and Go to File ? New ? New Project and enter your Application Name.
» Enter company domain, this is used to uniquely identify your App’s package worldwide. Remember to use the same package name as used in the firebase console.
» Choose project location and minimum SDK and on the next screen choose Empty Activity, Then Click on Next.
» Choose an Activity Name. Make sure Generate Layout File check box is selected, Otherwise, we have to generate it ourselves. Then click on Finish. We have used the Activity Name as MainActivity since this will be the default screen when the user opens the app for the first time.
Gradle will configure and build your project and resolve the dependencies, Once it is complete proceed for next steps.
Add Permissions and Dependencies
» After Gradle syncs the project, add the google-services.json file that you downloaded while creating a new project on the Firebase console to your project’s app folder as shown below. You can drag the file and put it inside the Android app module folder directly into android studio or you can simply copy the file and paste it inside the Android Studio Project App directory on your computer.
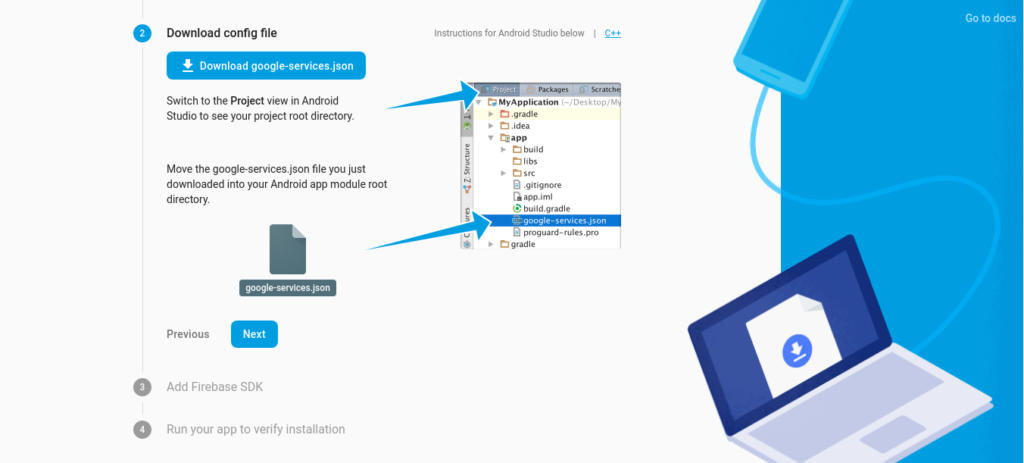
Since we need to connect to the Network while authenticating, you’ll need to add the Internet permission in your AndroidManifest.xml file which is more like a configuration file for your App.
AndroidManifest.xml file
3. open your project’s build. gradle file from the project’s home directory and add the following dependency. Please note that I’m not talking about the module or app’s build. gradle file, we’ll get to that in the next step
Build.gradle file
classpath ‘com.google.gms:google-services:3.0.0’
4. Next, open your app’s build.gradle from the and add the following at the end of the file.
apply plugin: ‘com.google.gms.google-services’
5. Also add the following dependency in the dependency section.
implementation ‘com.google.firebase:firebase-core:16.0.6’
implementation ‘com.google.firebase:firebase-auth:16.0.6’
implementation ‘com.google.android.gms:play-services-auth:16.0.1’
After adding these dependencies, Android Studio would want to sync, make sure you are connected to the internet so Android Studio can download these used dependencies.
1. Create Activity
Create activity_main.xml file in the res/layout folder and add the following code. We’ll just have only the Custom Google SignIn Button on this layout.
Now create MainActivity.java in the App/Java folder, create a method called configureGoogleApi, outside the onCreate() method and put in the below codes. then call that method in the onCreate method of the class.
we need to configure Google Sign-In in order to be able to get the user data. So, we create a GoogleSignInOptions object with the request email option.
private void configureGoogleApi() {
// Configure sign-in to request the user’s basic profile like name and email
GoogleSignInOptions options = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestEmail()
.build();
// Build a GoogleApiClient
mGoogleApiClient = new GoogleApiClient.Builder(this)
.enableAutoManage(this, this)//register callbacks for failure
.addApi(Auth.GOOGLE_SIGN_IN_API, options)// add Google Sign In Api with above created options
.build();
}
Next, set OnClickListener for signIn Button in onCreate(), this will prompt the user to select a Google account before the authentication process begins. this will handle sign-in by creating a sign-in intent with the getSignInIntent() method, and starting the intent with startActivityForResult().
binding.signIn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent signInIntent = Auth.GoogleSignInApi.getSignInIntent(mGoogleApiClient);
startActivityForResult(signInIntent, RC_SIGN_IN);
}
});
After the user selects an account and the process of authentication with Google begins, the result will be made available in the onActivityResult() as stated in the piece of code below. When the signIn button is clicked, the user will first be authenticated with Google and if that is successful, save user data to SharedPreference.
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
// Result returned from launching the Intent from GoogleSignInApi.getSignInIntent(…);
if (requestCode == RC_SIGN_IN) {
GoogleSignInResult result = Auth.GoogleSignInApi.getSignInResultFromIntent(data);
if (result.isSuccess()) {
// Google Sign In was successful
GoogleSignInAccount account = result.getSignInAccount();
if (account!=null) {
String idToken = account.getIdToken();
String name = account.getDisplayName();
String email = account.getEmail();
Uri photoUri = account.getPhotoUrl();
if (photoUri != null) {
String photo = photoUri.toString();
}
// Save Data to SharedPreference
}
} else {
// Handle Google Sign In failure
Toast.makeText(this, “Login Unsuccessful”, Toast.LENGTH_SHORT).show();
}
}
}
Below is the whole code of MainActivity for ease of integration.
public class MainActivity extends AppCompatActivity implements GoogleApiClient.OnConnectionFailedListener {
private static final int RC_SIGN_IN = 100;
private ActivityMainBinding binding;
private GoogleApiClient mGoogleApiClient;
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
binding = DataBindingUtil.setContentView(this, R.layout.activity_main);
configureGoogleApi();
binding.signIn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent signInIntent = Auth.GoogleSignInApi.getSignInIntent(mGoogleApiClient);
startActivityForResult(signInIntent, RC_SIGN_IN);
}
});
}
private void configureGoogleApi() {
// Configure sign-in to request the user’s basic profile like name and email
GoogleSignInOptions options = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestEmail()
.build();
// Build a GoogleApiClient
mGoogleApiClient = new GoogleApiClient.Builder(this)
.enableAutoManage(this, this)//register callbacks for failure
.addApi(Auth.GOOGLE_SIGN_IN_API, options)// add Google Sign In Api with above created options
.build();
}
@Override
public void onConnectionFailed(@NonNull ConnectionResult connectionResult) {
//handle failures
Log.e(“failed To Connect”,connectionResult.getErrorMessage()+””);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
// Result returned from launching the Intent from GoogleSignInApi.getSignInIntent(…);
if (requestCode == RC_SIGN_IN) {
GoogleSignInResult result = Auth.GoogleSignInApi.getSignInResultFromIntent(data);
if (result.isSuccess()) {
// Google Sign In was successful, save Token and a state then authenticate with Firebase
GoogleSignInAccount account = result.getSignInAccount();
if (account!=null) {
String idToken = account.getIdToken();
String name = account.getDisplayName();
String email = account.getEmail();
Uri photoUri = account.getPhotoUrl();
if (photoUri != null) {
String photo = photoUri.toString();
}
// Save Data to SharedPreference
}
} else {
// Handle Google Sign In failure, update UI appropriately
Toast.makeText(this, “Login Unsuccessful”, Toast.LENGTH_SHORT).show();
}
}
}
}
Now, run the app and click on Google Sign In Button to authenticate using Google Login. It should show a popup with available google account Click on your google account and the user is now authenticated using Google Sign In.
So, there you go. You can build the project and see that the google authentication is good to go.
Summing Up:
Following a few steps can give your project a google signup feature and will be beneficial and allow smooth processing for your customers. With this feature, you can definitely increase the number of registrations for your website or app.
We can help you to build many such interesting features for your app at Coruscate. Give us a call if you have an idea. We can help in shaping your ideas to reality.
» Android Debug Keystore SHA1 Fingerprint Tutorial :
Tutorial to Generating SHA-1
Certain Google Play services (such as Google Sign-in and App Invites) require you to provide the SHA-1 of your signing certificate so we can create an OAuth2 client and API key for your app. To get your SHA-1, follow these instructions:
Open a terminal and run the keytool utility provided with Java to get the SHA-1 fingerprint of the certificate. You should get both the release and debug certificate fingerprints.
To get the release certificate fingerprint:
keytool -exportcert -list -v \
-alias <your-key-name> -keystore <path-to-production-keystore>
To get the debug certificate fingerprint:
MAC/LINUX:
keytool -exportcert -list -v \
-alias androiddebugkey -keystore ~/.android/debug.keystore
WINDOWS:
keytool -exportcert -list -v \
-alias androiddebugkey -keystore %USERPROFILE%\.android\debug.keystore
The keytool utility prompts you to enter a password for the keystore. The default password for the debug keystore is android. The keytool then prints the fingerprint to the terminal. For example:
Certificate fingerprint: SHA1: DA:39:A3:EE:5E:6B:4B:0D:32:55:BF:EF:95:60:18:90:AF:D8:07:09
For more info visit:
https://developers.google.com/android/guides/client-auth