Benefits of Pairing React With Socket.IO to Build Super Real-time App
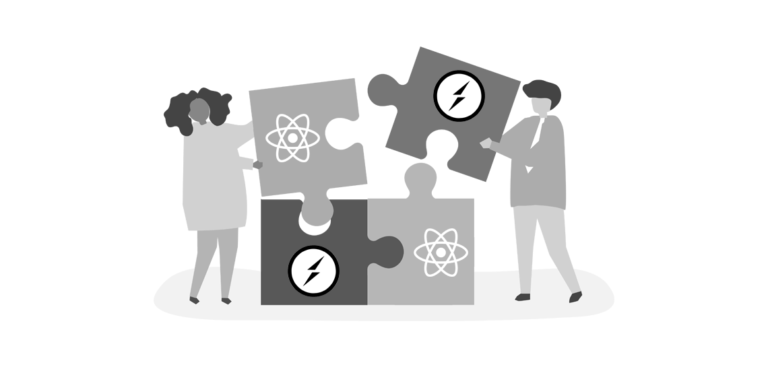
As a developer, It is really important to choose the right tech stack you will be going to deliver an app. And you need to make your app respond to events from the server side, which is referred to as real-time.
That means you don’t rely on the client side to decide when to get data from the server. Instead, the server will fetch data down to the client as it becomes available. Data gets pushed to the client from the server, instead of being pulled of being from the client.
This kind of model is well suited for a variety of applications such as chat, games, trading, etc. With the use of react and socket.io, more and more developers have started utilizing this case model where it is not needed for the product or app to work, but to make the app or product feel more responsive.
There are great benefits on how to use sockets and React to build your app more real-time. The article will show you the steps to develop a real-time app.
Must-Have Basics to Follow During Real-time App
On a very initial phase, you need to have the basics installed like node and npm (node package manager). In case if you don’t have that, you can install create-react-app to get started. This can be implemented by running the command like npm –global i create-react-app.
Next, you can proceed to generate the app and you need to play around with sockets by running the command: create-react-app socket-timer.
Once the app has created, you need to open the folder with text editor you like the most. To run it smoothly, you need to do is run npm start in the app folder.
Hosting Socket.io on the Server
Now you require to create a WebSocket service immediately. To complete this, drop into a terminal in created app folder and just install socket.io: npm i –save socket.io.
Now, socket.io is successfully installed, further you need to make a file named server.js in the root folder of the app which you build earlier. You can type the code const io = require(‘socket.io’)(); to import and construct the socket.
Here Websockets come into the role of long-running duplex channels between the client and server. The most essential thing to do on the server is to manage a client connection so that you can emit (publish) events to that client. Look the following syntax:
io.on(‘connection‘, (client) => {
// here you can start emitting events to the client
});
As soon as you’ve completed that, you also require to tell socket.io to start listening for clients.
const port = 8000;
io.listen(port);
console.log(‘listening on port ‘, port);
You can drop into terminal and start up the server by running node server. There should be a message that it started up successfully: listening on port 8000.
At the moment, the socket service is doing nothing. You have access to client sockets, still, you’re not publishing anything to them. While you have access to a connected client in this field, you can reply to events being emitted from the client. Consider of it as a server-side event handler concerning to a specific event from a specific client.
If you want the service to begin an interval (timer) and release the current date back to the client. You need the service to start a timer per client and the client can pass in the desired period of time.
It is an important point because it means that clients can send data via the server socket. You can change the code to add the following:
io.on(‘connection‘, (client) => {
client.on(‘subscribeToTimer’, (interval) => {
console.log(‘client is subscribing to timer with interval ‘, interval);
});
});
You have built up the basic structure to handle a client connection and for handling said client emitting an event to start a timer for it.
By that you can really start up the timer, and start emitting events back to the client including the current time, so you need to change the code as follows:
io.on(‘connection’, (client) => {
client.on(‘subscribeToTimer’, (interval) => {
console.log(‘client is subscribing to timer with interval ‘, interval);
setInterval(() => {
client.emit(‘timer’, new Date());
}, interval);
});
});
You’ve fired up a socket and began listening on it for clients. When a client connects, you have a closure where you can control events from a client. You can also handle a specific event, subscribeToTimer , being released from the client where you start a timer. When yosubscribeur timer fires, you emit an event, time , back to the client.
At this stage, the server.js file should look like this:
const io = require(‘socket.io’)();
io.on(‘connection’, (client) => {
client.on(‘subscribeToTimer’, (interval) => {
console.log(‘client is subscribing to timer with interval ‘, interval);
setInterval(() => {
client.emit(‘timer’, new Date());
}, interval);
});
});
const port = 8000;
io.listen(port);
console.log(‘listening on port ‘, port);
That’s done for the server side from socket.io setup! Now you can move on to the client side. Before that make sure that you have latest server code running by running node server in a terminal. If you started this up before you did your latest changes, just restart it.
Hosting Socket.io On the Client:
As you already began with React app earlier by running npm start on the command line. Then you should be able to go into the code, make changes, and see the browser reloading your app with the changes.
Now you need to is wire up the client sockets code that will communicate with the server side socket to start a timer, by emitting subscribeToTimer, and use the timer events emitted through the server.
Make a file in the src folder named api.js to make this hapen. In the api.js file, you require to create a function that can be called to emit the subscribeToTimer event to the server and feedback the completion of the timer event to the consuming code.
You can define the function, and exporting it from the module:
function subscribeToTimer(interval, cb) {
}
export { subscribeToTimer }
If you are basically opting to use a node style function, where the caller of this function can transfer in an interval for the timer on the first parameter, and a callback function on the second parameter.
You need to install the socket.io client library, if you want to communicate to the socket on the server. You can do on the command line, install npm: npm –save socket.io-client. You can also create the socket by calling the main export function from the socket.io-client module, providing a port, which in case is 8000.
import openSocket from ‘socket.io-client’;
const socket = openSocket(‘http://localhost:8000’);
On the server side, you have to wait for a client to emit the subscribeToTimer event to the server before starting a timer and then emit the timer event to the client every time the timer reports.
First, all you need to do now is to subscribe to the timer event coming from the server and then emit the subscribeToTimer event. Every time you receive a timer event from the server.
How to Apply the Events in a React Component?
You must have a file named api.js on the client side that exports a function that you can call to subscribe to timer events. Following, you’ll understand how you can use the function in your React component to perform the timer value every time the server emits it.
At the top of the App.js file it was built by create-react-app, import the api that we created in beginning.
import { subscribeToTimer } from ‘./api’;
Almost you have achieved that, you can proceed ahead and add a constructor for the component and inside of the constructor, call the subscribetoTimer function that you see from the api file. Every time that you receive an event, make sure to set a value called timestamp on state using the value that came through from the server.
constructor(props) {
super(props);
subscribeToTimer((err, timestamp) => this.setState({
timestamp
}));
}
As you will be going to use the timestamp value on state, it makes sense to include a default value for it. You can add the bit of code just below the constructor to set some default state:
state = {
timestamp: ‘no timestamp yet’
};
Further, you can now update your render function to render the timestamp you’ve placed on state in the event handler.
render() {
return (
);
}
After performing many steps, you are able to see the events coming from the server and being distributed inside of your React component. However, there is quite a little more to developing real-time apps with React and Socket.io.
The above post is inspired by one of the online resources and here we have tried to explain it with adding our thoughts in it to ease your job. You can contact us if you require the assistance of socket.io services to integrate with top technologies to create real-time application.
Source: (https://medium.com/dailyjs/combining-react-with-socket-io-for-real-time-goodness-d26168429a34)